This page provides typical use cases for dynamic site messaging along with the JavaScript scripts for implementing them.
Note: The scripts are triggered on specific pages and / or in emails and can be conditional in nature. For an overview of setting up dynamic site messaging, see Dynamic Site Messaging.
All trips
Multi-city price changes
Any multi-city itinerary triggers a message to display on the designated pages and / or emails.
| | |
---|
DSM | Multi-City Price Changes |
//Multi Destination
var md=UserPnrData.TravelType;
if (md=="MultiDest") {out.print("true");}
else {out.print("false");}
|
Itinerary is awaiting pre-trip approval
All itineraries awaiting pre-trip approval trigger a message to display on the designated pages and / or emails.
| | |
---|
DSM | Awaiting Pre-trip Approval |
//AWAITING PRE-TRIP APPROVAL
if (UserPnrData.UserBooking.PreTripApprovalInfo.CurrentApprovalState=="WAITING_FOR_APPROVAL")
{out.print("true");}else
{out.print("false");}
|
Travel requested between dates to specified city
Travel requested for specified date range to a specified city triggers a message on the designated pages.
| | |
---|
DSM | Travel Date Range to Specified City |
//Travel Request Between Dates to CityCode
//checks if input dateToCheck is between - "startDateRange" and "endDateRange" (inclisive)
function isDateBetween(dateToCheck, startDateRange, endDateRange) {
var df = new java.text.SimpleDateFormat('yyyy-MMM-dd');
var d = df.parse(dateToCheck);
var min = df.parse(startDateRange);
var max = df.parse(endDateRange);
//inclusive date range check
return d.compareTo(min) >= 0 && d.compareTo(max) <= 0;
}
//return date in format - "YYYY-MMM-DD" e.g. '2016-MAR-01'
function formattedDate(flightLeg) {
if (flightLeg) {
var year = flightLeg.DepartureDate.Year;
var month = flightLeg.DepartureDate.Month;
var day = flightLeg.DepartureDate.Day;
return year + '-' + month + '-' + day;
}
}
//get air travel "start date"
function getAirStartDate() {
return formattedDate(UserPnrData.UserSession.AirSearch.FlightLeg[0]);
}
//get air travel "end date"
function getAirEndDate() {
return formattedDate(UserPnrData.UserSession.AirSearch.FlightLeg[1]);
}
var startDate = getAirStartDate();
var endDate = getAirEndDate();
//By using subscript "FlightLeg[0]", we can access first leg,
//"FlightLeg[1]" gives second leg and so on...
//In this case, we are checking if destination of first leg is JFK (or not).
var isAirportJFK = (UserPnrData.UserSession.AirSearch.FlightLeg[0].DestinationAirportCode == 'JFK');
//as an example, we will check "startDate"
//please replace below code with actual date you would like to check
//date ranges have to be written in format - YYYY-MMM-DD for e.g. 2016-MAR-01
if (startDate) {
var isBetween = isDateBetween(startDate, '2016-MAR-01', '2016-MAR-24');
out.print(isBetween && isAirportJFK);
} else {
out.print('false');
}
|
Profile field contains specific value
If a traveler profile contains the specified value, a message displays on the designated pages and / or emails. For example, if the profile contains Business Unit 789 or if the user has a Known Traveler Number/Redress Number, then a message could display.
| | |
---|
DSM | Specific Value in Profile Field |
//Profile Element
if (UserPnrData.User.UserInfo.BusinessUnit=="789")
{out.print("true");}else
{out.print("false");}
//Profile Element
if ((UserPnrData.User.AirlinePreferences.KnownTravelerNumber != undefined)||(UserPnrData.User.AirlinePreferences.RedressNumberSet.RedressNumber != undefined))
{out.print("true");}
else {out.print("false");}
|
Mobile push notifications
Trigger the system to send mobile push notifications to travelers via the mobile app (not email or text based).
Tag/keyword | Secondary tag/ keywords | Script |
---|
DSM | Mobile Push Notifications |
var display = "false";
if ((UserPnrData.UserBooking.HotelBooking == "")&&(UserPnrData.UserBooking.AirBooking != "")&&(UserPnrData.MeetingInfo.MeetingHeader.MeetingID == ""))
{
|
DSM for agency office hours
Tag/Keyword | Secondary tag/keywords | Script |
---|
DSM | Agency Office Hours |
var min=mydate.getMinutes()
{if ((today==0) || (today==6) || (hours < 11 && min >30)||(hours > 17))
{display = "true";} else {display = "false";}}
}
out.print(display);
|
Flights
| | |
---|
DSM | Covid Booking Alert |
<br><p><span style="font-size: medium;">Are you booking international travel? Check the Travel Advisories to learn about your destination. <a href="https://travel.state.gov/content/travel/en/traveladvisories/traveladvisories.html/." target="_blank" rel="noopener">Learn more</a></span></p>
<p><span style="font-size: medium;"><br /><br /> CDC: Considerations for Travelers—Coronavirus in the US. <a href="https://www.cdc.gov/coronavirus/2019-ncov/travelers/travel-in-the-us.html" target="_blank" rel="noopener">Learn more</a></span></p>
|
Duplicate booking alert
All reservations tagged as being duplicates of previously booked reservations trigger a message to display on the designated pages and / or emails.
| | |
---|
DSM | Duplicate Booking Alert |
//DUPLICATE BOOKING ALERT
var dupe=UserPnrData.UserBooking.HasOverlappingFlights;
if (dupe=="Y") {out.print("true");}
else {out.print("false");}
|
Selected carrier is in list
All search results and / or reservations for the requested carrier trigger a message to display on the designated pages and / or emails.
| | |
---|
DSM | Selected Carrier Is In List |
//Selected Carrier is in List
if ((((UserPnrData.UserBooking.AirBooking.FlightLeg[0].FlightSegment[0].CarrierCode=="LH")||(UserPnrData.UserBooking.AirBooking.FlightLeg[0].FlightSegment[0].CarrierCode=="AC")||(UserPnrData.UserBooking.AirBooking.FlightLeg[0].FlightSegment[0].CarrierCode=="DL")||(UserPnrData.UserBooking.AirBooking.FlightLeg[0].FlightSegment[0].CarrierCode=="UA"))))
{out.print("true");}else
{out.print("false");}
|
Contains an international segment
All reservations containing an international segment trigger a message to display on the designated pages and / or emails.
If message is to be displayed prior to the user having SELECTED an international flight (that is, the flight search results page), DO NOT add script but ensure radio button "International trips only" under “Trip type to show message" has been selected.
| | |
---|
DSM | Contains an International Segment |
//INTERNATIONAL SEGMENT
var Int=UserPnrData.UserBooking.HasInternationalSegment;
if (Int=="Y") {out.print("true");}
else {out.print("false");}
|
Contains a voidable ticket
All searches and / or reservations containing a voidable ticket trigger a message to display on the designated pages and / or emails.
| | |
---|
DSM | Contains a Voidable Ticket |
//VOIDABLE TICKET
if (UserPnrData.TicketStatus.Ticket.Action=="void")
{out.print("true");}else
{out.print("false");}
|
Unused ticket on file offered
User selected flights and an unused ticket on file has been offered for the option.
Sample use case: display message advising user how to opt out of unused ticket (if enabled in Display Configuration). For example, if you would rather not apply an unused ticket offered for this trip, click the x to the right of the "Unused Ticket" reference within the Trip Cost Summary box.
| | |
---|
DSM | Unused Ticket Offered |
//UNUSED TICKET OFFERED
if (UserPnrData.UserBooking.UnusedTicketUsage[0].TicketNumber != undefined)
{out.print("true");}else
{out.print("false");}
|
Frequent flyer number for specified carrier
All searches and / or reservations where a frequent flyer number is present for a specified carrier triggers a message to display on the designated pages and / or emails.
| | |
---|
DSM | Frequent Flyer Number for Carrier In List |
//FREQUENT FLYER NUMBER PRESENT FOR CARRIER IN LIST
var myFFnumber=UserPnrData.User.AirlinePreferences.MembershipSet.Membership;
var i=0;
var j=0;
var ifToPrint = "false";
for (var i = 0; i < myFFnumber.length(); i++) {
with (UserPnrData.User.AirlinePreferences.MembershipSet.Membership[i]) {
if (AirlineCode == "UA"){
ifToPrint="true";
}
}
}
out.print(ifToPrint);
|
Flight cost
Flights greater then the specified cost triggers a message on the designated pages.
| | |
---|
DSM | Flight Cost |
//Air Ticket Cost
var fare=UserPnrData.UserBooking.AirBooking.AirFare.FareInfo.TotalFare;
if (fare>700.00)
{out.print("true");}else
{out.print("false");}
|
Flight is out of policy
Out of policy flights trigger a message on the designated pages.
| | |
---|
DSM | Flight Out of Policy |
//Out of policy
var OOP=UserPnrData.UserSession.ComplianceCodes.AirCode;
if (OOP == undefined) {out.print("false");}
else {out.print("true");}
|
Carrier is in list
Flights for specified carriers trigger a message on the designated pages.
| | |
---|
DSM | Air Carrier is In List |
//SELECTED CARRIER IS IN LIST
if (UserPnrData.UserBooking.AirBooking.FlightLeg[0].FlightSegment[0].CarrierCode=="AA")
{out.print("true");}else
{out.print("false");}
|
Fare tier = UNMODIFIABLE (characteristic of BASIC FARE TIER)
A fare option where the fare details include "Fare Tier" description of "UNMODIFIABLE", generally indicates that it's a Basic Fare. A message can be triggered based on this description, on the designated pages.
| | |
---|
DSM | FareTier is UNMODIFIABLE |
//BASIC FARE TIER
if (UserPnrData.UserBooking.AirBooking.AirFare.FareInfo.FareTier=="UNMODIFIABLE")
{out.print("true");}else
{out.print("false");}
|
Delta E-Fare
Tag/keyword | Secondary tag/keywords | Script |
---|
DSM | Delta E-Fares |
var ifToPrint = "false";
with (UserPnrData.UserBooking.AirBooking) {
for (var iFL=0; iFL<FlightLeg.length(); iFL++) {
for (var iFS=0; iFS<FlightLeg[iFL].FlightSegment.length(); iFS++) {
if ((FlightLeg[iFL].FlightSegment[iFS].CarrierCode == "DL")&&
(FlightLeg[iFL].FlightSegment[iFS].BookingCode == "E"))
{
ifToPrint = "true";
break;
}
}
}
}
out.print(ifToPrint);
|
Class of service selected
Specified classes of service trigger a message on the designated pages.
| | |
---|
DSM | Class of Service |
//Cabin Class
var ifToPrint = "false";
with (UserPnrData.UserBooking.AirBooking) {
for (var iFL=0; iFL<FlightLeg.length(); iFL++) {
for (var iFS=0; iFS<FlightLeg[iFL].FlightSegment.length(); iFS++) {
if (FlightLeg[iFL].FlightSegment[iFS].ServiceClass == "Coach") {
ifToPrint = "true";
break;
}
}
}
}
out.print(ifToPrint);
|
Class of service searched
Specified classes of service trigger a message on the designated pages.
| | |
---|
DSM | Class of Service | //FIRST OR BUSINESS CABIN CLASS SEARCHED
var ifToPrint = "false";
{ var myFlightLeg=UserPnrData.UserSession.AirSearch.FlightLeg; var numLegs=myFlightLeg.length(); var i=0; for (i=0; i<numLegs; i++)
with (UserPnrData.UserSession.AirSearch.FlightLeg[i])
{if ( (ServiceClass == "first") || (ServiceClass == "business") )
{ ifToPrint = "true";
break; }
} } out.print(ifToPrint); |
14 day required advance ticketing
Specified advance dates trigger a message on the designated pages.
Tag/keyword | Secondary tag/keywords | Script |
---|
DSM | Advanced Ticketing |
var ifToPrint="false";
var Month1=
UserPnrData.UserBooking.AirBooking.FlightLeg[0].FlightSegment[0].DepartureDate.Month;
var Day1=
UserPnrData.UserBooking.AirBooking.FlightLeg[0].FlightSegment[0].DepartureDate.Day;
var Year1=
UserPnrData.UserBooking.AirBooking.FlightLeg[0].FlightSegment[0].DepartureDate.Year;
var Month2=UserPnrData.UserBooking.AgencyTransactionDate.Month;
var Day2=UserPnrData.UserBooking.AgencyTransactionDate.Day;
var Year2=UserPnrData.UserBooking.AgencyTransactionDate.Year;
var date1=new Date(Month1 + " " + Day1 + ", " + Year1);
var date2=new Date(Month2 + " " + Day2 + ", " + Year2);
var days=0;
var difference=0;
difference=date1-date2;
days = difference/(1000*60*60*24);
if((UserPnrData.UserBooking.AirBooking!=undefined)&&(days<14)){
ifToPrint="true";}
out.print(ifToPrint);
|
Specified origination and destination airport searched
Specified originating airport and destination airport SEARCHED triggers a message on the designated pages.
| | |
---|
DSM | Origin and Destination Airport searched is In List |
//ORIGIN AND DESTINATION AIRPORTS SPECIFIED SEARCHED
var ifToPrint = "false";
{
var myFlightLeg=UserPnrData.UserSession.AirSearch.FlightLeg;
var numLegs=myFlightLeg.length();
var i=0;
for (i=0; i<numLegs; i++)
with (UserPnrData.UserSession.AirSearch.FlightLeg[i])
{if (((
((OriginAirportCode == "LGA") && (DestinationAirportCode == "ORD")) ||
((OriginAirportCode == "ORD") && (DestinationAirportCode == "LGA")) ||
(OriginAirportCode == "LAX") && (DestinationAirportCode == "ORD")) ||
(OriginAirportCode == "ORD") && (DestinationAirportCode == "LAX"))
)
{ ifToPrint = "true";
break; }
} }
out.print(ifToPrint);
|
Specified origination airport selected
Specified originating airport selected triggers a message on the designated pages.
| | |
---|
DSM | Origin Airport is In List |
//Origin Airport Code
if (UserPnrData.UserSession.AirSearch.FlightLeg[0].OriginAirportCode=="ORD")
{out.print("true");}else
{out.print("false");}
|
Specified origination and destination airport selected
Specified originating airport selected triggers a message on the designated pages.
| | |
---|
DSM | Origin and Destination Airport is In List |
//ORIGIN AND DESTINATION AIRPORTS SPECIFIED
var ifToPrint = "false";
{
var myFlightLeg=UserPnrData.UserBooking.AirBooking.FlightLeg;
var numLegs=myFlightLeg.length();
var i=0;
for (i=0; i<numLegs; i++)
with (UserPnrData.UserBooking.AirBooking.FlightLeg[i])
{if (((
((OriginAirportCode == "LGA") && (DestinationAirportCode == "ORD")) ||
((OriginAirportCode == "ORD") && (DestinationAirportCode == "LGA")) ||
(OriginAirportCode == "LAX") && (DestinationAirportCode == "ORD")) ||
(OriginAirportCode == "ORD") && (DestinationAirportCode == "LAX"))
)
{ ifToPrint = "true";
break; }
} }
out.print(ifToPrint);
|
Hurricane Irma disaster recovery - guide travelers to change flight reservation
Place static messaging on the site guiding travelers to change their reservations online if possible (that is, if change/cancel enabled) or to call a non-emergency agency number if their travel is not within X hours. This message will lessen those calls for non-immediate assistance and allow the agents to focus on travelers with more immediate travel needs.
| | |
---|
DSM | Disaster Airport is In List |
<b><font color="red" size="4">URGENT – HURRICANE EMERGENCY PROCEDURES<BR><BR></font>
<font color="red" size="3">Please use our COMPANY travel site for any changes/cancellations if at all possible!<BR>
Due to emergency hurricane efforts, please limit calls for travel agent support unless travelling in the next 72 hours. </b></font>
|
For Example:
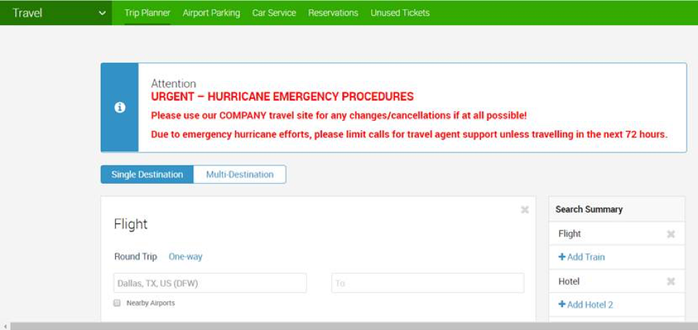
Hurricane Irma disaster recovery - display messaging for 11 Florida airport searches
Use our Dynamic Site Messaging functionality to display pertinent messaging when travelers are searching for flights to any of the top 11 FL airports.
| | |
---|
DSM | Disaster Airport is In List |
<b><font color="red" size="4">FLORIDA FLIGHT PROVISIONS HURRICANE IRMA:<BR><BR></font>
<font color="red" size="3">COMPANY out-of-policy notifications have been suspended for flights to or from Florida within the next 72 hours.<BR><BR> Our travel agents can assist you as needed, but it is not necessary to call for travel policy questions during this time. Please anticipate and excuse longer hold times during this time.</b></font>
JAVA for above messaging to fire when searching for any of the 11 major airports in FL
//irma
var ifToPrint="false";
{var pul=UserPnrData.UserSession.AirSearch.FlightLeg[0].OriginAirportCode;
if(((pul=="MCO")||(pul=="MIA")||(pul=="FLL")||(pul=="PBI")||(pul=="RSW")||(pul=="APF")||(pul=="SRQ")||(pul=="TPA")||(pul=="JAX")||(pul=="TLH")||(pul=="PNS")) && (UserPnrData.UserBooking.AirBooking != "")){
ifToPrint="true";
}
out.print(ifToPrint);}
|
For Example:
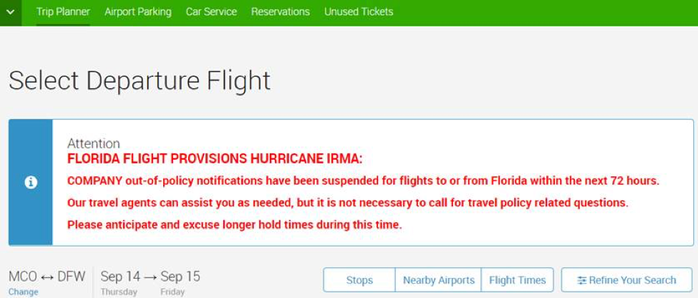
Hotel
| | |
---|
DSM | Covid Booking Alert |
<br><p><span style="font-size: medium;">Understand your risks when staying in a hotel. Take certain precautions and follow social distancing guidelines. <a href="https://www.goodhousekeeping.com/health/a32859542/is-it-safe-to-stay-hotels-coronavirus/" target="_blank" rel="noopener">Learn more</a></span></p>
<p><span style="font-size: medium;"><br /><br /> Be prepared for your trip. See what experts say about hotels and CDC guidelines for COVID-19. <a href="https://www.healthline.com/health-news/covid-19-hotels-airbnb-safety" target="_blank" rel="noopener">Learn more</a></span></p>
|
Hotel search at specified airport, city name, and/or state
All searches for a hotel at a specific location trigger a message to display on the designated pages.
| | |
---|
DSM | Hotel Search at Airport, City Name and/or State In List |
//HOTEL SEARCH AT SPECIFIED AIRPORT CITY and or STATE
if
(
(UserPnrData.UserSession.HotelSearch.Hotel.AirportCode=="SJC") ||
(UserPnrData.UserSession.HotelSearch.Hotel.Address.City=="San Jose") ||
(UserPnrData.UserSession.HotelSearch.Hotel.Address.City=="Sunnyvale") ||
(UserPnrData.UserSession.HotelSearch.Hotel.Address.State=="AK") ||
((UserPnrData.UserSession.HotelSearch.Hotel.Address.City=="Newark") && (UserPnrData.UserSession.HotelSearch.Hotel.Address.State=="DE"))
)
{out.print("true");}else
{out.print("false");}
|
Hotel search for San Francisco
All searches for San Francisco hotels trigger a message to display on the designated pages. A San Francisco city search provides different results on the back-end than other searches, requiring a slightly different script than the standard hotel location search script.
| | |
---|
DSM | Hotel Search for San Francisco |
//HOTEL SEARCH FOR SAN FRANCISCO
if
((((UserPnrData.UserSession.HotelSearch.Hotel.AirportCode=="SFO") || (UserPnrData.UserSession.HotelSearch.Hotel.City=="SF") || (UserPnrData.UserSession.HotelSearch.Hotel.Address.City.startsWith(“SF") ||(UserPnrData.UserSession.HotelSearch.Hotel.City=="San Francisco"))))
{out.print("true");}else
{out.print("false");}
|
Hotel chain is in list
Specific hotel chain selected triggers a message to display on the designated pages.
| | |
---|
DSM | Hotel Chain is In List |
//SELECTED HOTEL CHAIN IS IN LIST
if (UserPnrData.UserBooking.HotelBooking.Hotel[0].HotelChainCode=="HH")
{out.print("true");}else
{out.print("false");}
|
Hotel selection is out of policy
Out of policy hotel selection triggers a message to display on the designated pages.
| | |
---|
DSM | Hotel is Out of Policy |
//OUT OF POLICY HOTEL
var OOP=UserPnrData.UserSession.ComplianceCodes.HotelCode;
if (OOP == undefined) {out.print("false");}
else {out.print("true");}
|
14 day advance notice needed for hotels
Any hotel reservation with less than 14 days advance notice triggers this message.
Tag/keyword | Secondary tag/keywords | Script |
---|
DSM | 14 day advance notice |
var ifToPrint="false";
var Month1=
UserPnrData.UserBooking.HotelBooking.Hotel[0].Room.CheckInDate.Month;
var Day1=
UserPnrData.UserBooking.HotelBooking.Hotel[0].Room.CheckInDate.Day;
var Year1=
UserPnrData.UserBooking.HotelBooking.Hotel[0].Room.CheckInDate.Year;
var Month2=UserPnrData.UserBooking.AgencyTransactionDate.Month;
var Day2=UserPnrData.UserBooking.AgencyTransactionDate.Day;
var Year2=UserPnrData.UserBooking.AgencyTransactionDate.Year;
var date1=new Date(Month1 + " " + Day1 + ", " + Year1);
var date2=new Date(Month2 + " " + Day2 + ", " + Year2);
var days=0;
var difference=0;
difference=date1-date2;
days = difference/(1000*60*60*24);
if((UserPnrData.UserBooking.HotelBooking.Hotel!=undefined)&&(days<14)){
ifToPrint="true";}
out.print(ifToPrint);
|
No hotel is selected for overnight trip
Overnight trip not including a hotel triggers a message to display on the designated pages.
| | |
---|
DSM | No Hotel for Overnight Trip |
//No Hotel Booked
var display = "false";
if ((UserPnrData.UserBooking.HotelBooking == "")&&(UserPnrData.UserBooking.AirBooking != "")&&(UserPnrData.MeetingInfo.MeetingHeader.MeetingID == ""))
{
var myFlightLeg=UserPnrData.UserBooking.AirBooking.FlightLeg;
var numLegs=myFlightLeg.length();
var i=0;
var j=0;
var myFlightSeg;
var startDay = UserPnrData.UserBooking.AirBooking.FlightLeg[0].FlightSegment[0].DepartureDate.Day;
for (i=0; i<numLegs; i++) {
myFlightSeg=UserPnrData.UserBooking.AirBooking.FlightLeg[i].FlightSegment;
var numSegs=myFlightSeg.length();
for (j=0; j< numSegs; j++) {
with (UserPnrData.UserBooking.AirBooking.FlightLeg[i].FlightSegment[j]) {
if (startDay != DepartureDate.Day) {
display = "true";
}
else{
display = "false";
}
}
}
}
}
out.print (display);
|
Non-refundable hotel room type selected
A non-refundable room type selection triggers a message to display on the designated pages.
| | |
---|
DSM | Non Refundable |
//NON-REFUNDABLE HOTEL ROOM TYPE
if (((UserPnrData.UserBooking.HotelBooking.Hotel[0].Room[0].PrePaid!="false")||(UserPnrData.UserBooking.HotelBooking.Hotel[0].Room[0].CancellationPolicy=="00N")||(UserPnrData.UserBooking.HotelBooking.Hotel[0].Room[0].CancellationPolicy=="NOR")))
{out.print("true");}else
{out.print("false");}
|
Hotel room type selected
Specific hotel room type selection triggers a message to display on the designated pages.
| | |
---|
DSM | Hotel Room Type Selected |
//SELECTED HOTEL ROOM TYPE
if (UserPnrData.UserBooking.HotelBooking.Hotel[0].Room.RoomTypeCode=="King")
{out.print("true");}else
{out.print("false");}
|
Hotel loyalty number not in profile
A missing hotel loyalty number triggers a message to display on the designated pages.
| | |
---|
DSM | Hotel Loyalty Number Missing in Profile |
//Hotel FF NOT in profile
var myFFnumber=UserPnrData.User.HotelPreferences.MembershipSet.Membership;
var i=0;
var j=0;
var ifToPrint = "true";
for (var i = 0; i < myFFnumber.length(); i++) {
with (UserPnrData.User.HotelPreferences.MembershipSet.Membership[i]) {
if (ChainCode == "EH"){
ifToPrint="false";
}
}
}
out.print(ifToPrint);
|
Hotel rate change
A hotel with different nightly rates triggers a message to display on the designated pages.
| | |
---|
DSM | Hotel Rate Change |
if (UserPnrData.UserBooking.HotelBooking.Hotel.Room.RateChange=="true")
{out.print ("true");}else
{out.print("false");}
|
Hotel booked from Booking.com
Will trigger a message if confirmed hotel rate was sourced from Booking.com (post-purchase).
| | |
---|
DSM | Hotel Booking from Booking.com |
//SELECTED HOTEL FROM BOOKING.COM
if (UserPnrData.UserBooking.HotelBooking.Hotel.Room.RecordLocator.ProviderName=="BOOKING_DOT_COM")
{out.print("true");} else
{out.print("false");}
|
No hotel searched
A message triggers when no hotel is searched for (rather than booked).
Tag/keyword | Secondary tag/keywords | Script |
---|
DSM | No Hotel Searched |
//No Hotel Requested
if ((UserPnrData.UserSession.HotelSearch=="")||(UserPnrData.UserSession.HotelSearch==null))
{out.print("true");}else
{out.print("false");}
|
Hotel booked
Will trigger a message only if a hotel was booked (i.e., only display message on Review Trip or Purchase Page if a hotel was booked).
| | |
---|
DSM | Hotel Booking Exists |
//HOTEL BOOKING EXISTS
if (UserPnrData.UserBooking.HotelBooking !="")
{out.print("true");}else
{out.print("false");}
|
Message scrolls from right to left.
| | |
---|
DSM | Hotel Scrolling Message |
<font size="4" color="red"><marquee behavior="scroll" direction="left" scrollamount="7">Please look at hotel cancellation policies for refundable/deposits. </font></marquee>
|
Rental car
Display if car searched
Triggers a message only if a search was done for a rental car (i.e., only display message on Review Trip or Purchase Page if a car search).
| | |
---|
DSM | Rental Car was searched |
//Car Searched
{if(UserPnrData.UserSession.CarSearch!="")
{out.print("true");}else
{out.print("false");}}
|
One-way rental is searched
Triggers a message only if a one-way rental car is searched (i.e., only display message on Review Trip or Purchase Page if a car search).
| | |
---|
DSM | Rental Car was searched |
var PU=''+UserPnrData.UserBooking.CarBooking.Car.PickupLocation;
var DO=''+UserPnrData.UserBooking.CarBooking.Car.DropoffLocation;
if (PU === DO) {out.print("false");}
else {out.print("true");}
|
Car pick-up location is in list
Specific rental car company selected triggers a message to display on the designated pages.
| | |
---|
DSM | Rental Car Company is In List |
//Car Pick Up Location
var ifToPrint="false";
{var pul=UserPnrData.UserBooking.CarBooking.Car.PickupLocation;
if(((pul=="ANC")||(pul=="SIT")||(pul=="BET")||(pul=="FBK")||(pul=="ENA")||(pul=="JNU")||(pul=="KTN"))&& (UserPnrData.UserBooking.CarBooking != "")){
ifToPrint="true";
}
out.print(ifToPrint);}
|
14 day advance notice needed
Any car reservation with less than 14 days advance notice triggers this message.
Tag/keyword | Secondary tag/keywords | Script |
---|
DSM | 14 day advance |
var ifToPrint="false";
var Month1=
UserPnrData.UserBooking.CarBooking.Car[0].PickupDate.Month;
var Day1=
UserPnrData.UserBooking.CarBooking.Car[0].PickupDate.Day;
var Year1=
UserPnrData.UserBooking.CarBooking.Car[0].PickupDate.Year;
var Month2=UserPnrData.UserBooking.AgencyTransactionDate.Month;
var Day2=UserPnrData.UserBooking.AgencyTransactionDate.Day;
var Year2=UserPnrData.UserBooking.AgencyTransactionDate.Year;
var date1=new Date(Month1 + " " + Day1 + ", " + Year1);
var date2=new Date(Month2 + " " + Day2 + ", " + Year2);
var days=0;
var difference=0;
difference=date1-date2;
days = difference/(1000*60*60*24);
if((UserPnrData.UserBooking.HotelBooking!=undefined)&&(days<14)){
ifToPrint="true";}
out.print(ifToPrint);
|
Car size is in list
Specific car size selected triggers a message to display on the designated pages.
| | |
---|
DSM | Car Size is In List |
//SELECTED CAR SIZE
if (UserPnrData.UserBooking.CarBooking.Car[0].CarSize=="I")
{out.print("true");}else
{out.print("false");}
|
Car vendor is in list
Specific car vendor selected triggers a message to display on the designated pages.
| | |
---|
DSM | Car Vendor is In List |
//SELECTED CAR VENDOR IS IN LIST
if (UserPnrData.UserBooking.CarBooking.Car[0].VendorCode=="ZE")
{out.print("true");}else
{out.print("false");}
|
Car membership number is in profile
Profile car membership number triggers a message to display on the designated pages.
| | |
---|
DSM | Car Membership Number is in Profile |
//Car FF PRESENT FOR Vendor
var myFFnumber=UserPnrData.User.CarRentalPreferences.MembershipSet.Membership;
var i=0;
var j=0;
var ifToPrint = "false";
for (var i = 0; i < myFFnumber.length(); i++) {
with (UserPnrData.User.CarRentalPreferences.MembershipSet.Membership[i]) {
if (CompanyCode == "EH"){
ifToPrint="true";
}
}
}
out.print(ifToPrint);
|
Car selection is out of policy
A car selected car out of policy car triggers a message to display on the designated pages.
| | |
---|
DSM | Car is Out of Policy |
//Out of Policy Car
var OOP=UserPnrData.UserSession.ComplianceCodes.CarCode;
if (OOP == undefined) {out.print("false");}
else {out.print("true");}
|